2 Quick Tips I’ve learned for FE Testing as a LogicGate Dev
By: Kat Kodes | Updated on: May 27, 2020
Debugging is an essential part of writing code. During my time here at LogicGate I’ve learned a ton of helpful tips and tricks (big shout out to my teammates!) that make debugging on the Frontend more efficient. Below, I share two of my favorite debugging tips that have saved me time and headache.
Tip #1: Run a Single Test in Jasmine
We have almost 800 Frontend unit tests for our code base. You can imagine not wanting to have to run the entire test suite when adding a new test or updating an existing one. Luckily we use Jasmine and the library has a pretty simple — but not obvious — way to handle this.
The solution here? A simple f
! That is correct, all you need to do is put an f
in front of a describe
or it
block.
Here’s a block of test code:
describe('Component: Table Report', () => { it('should render', () => { expect(component).toBeDefined(); }); });
To run the entire block, you would just use fdescribe
:
fdescribe('Component: Table Report', () => { it('should render', () => { expect(component).toBeDefined(); }); });
To run an it
block individually you can also use fit
:
describe('Component: Table Report', () => { fit('should render', () => { expect(component).toBeDefined(); }); });
Simple, right? One caveat here is that if you run a single test this way, it is easy to forget to remove the f
from your code. The dev team at LogicGate has handled this by creating a linting rule that disallows fdescribe
and fit
as function names, and running our linter as part of our CI pipeline.
Tip #2: Add Breakpoints Using Chrome Dev Tools
(Note: I am using Google Chrome in all of the following screenshots. Other browsers may have similar functionality, but for the purpose of this blog I will only discuss Chrome.)
While our reliable friend console.log
will always be there when we need them, adding breakpoints adds more debugging functionality and access. I’ve used debugger
statements in my code to trigger a breakpoint using Chrome + Chrome Dev Tools in the past, but a fellow teammate showed me an easy way to add them directly in the browser.
To find the source file you want to place a breakpoint in, using the file name, open Chrome Dev Tools and go to the Sources
tab.

From the Sources
tab, use cmd + p
on a mac or ctrl + p
on a PC to open the file name search bar to then search for your file.
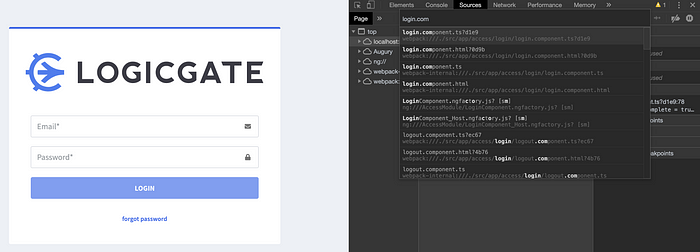
Once you’re in the file you want to place a breakpoint in, you can search within the file using cmd + f
or ctrl + f
. Place your breakpoint in the left column which shows the line numbers.
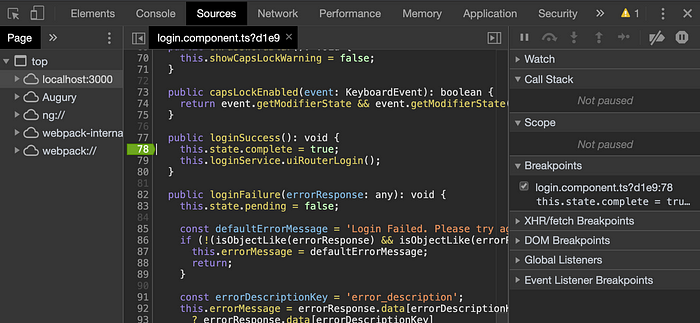
Next, interact with the the app in your browser to trigger the break point!
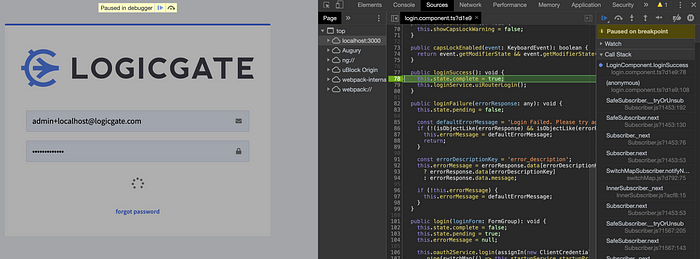
Once you hit the breakpoint, you’ll have access to any variables in the scope of the break point, and you can click through the steps of your code.
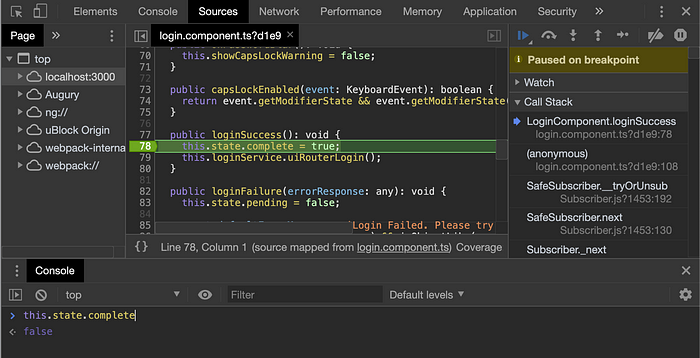
You can use the toolbar on the right to walk through code, play through your breakpoint, or disable breakpoints.

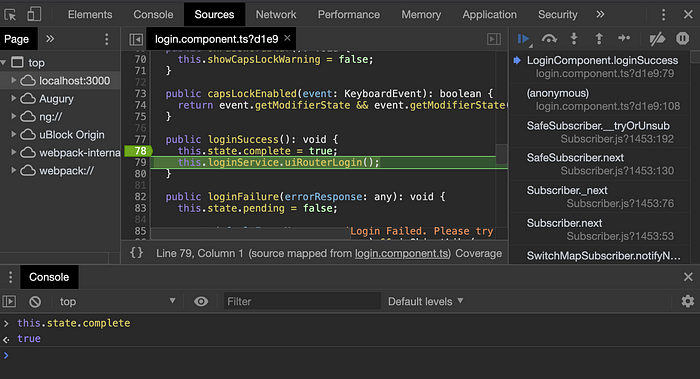
Wishing you the best on your testing endeavors.